Node.js & Express.js Series: Chapter 20 - Fetch records from the database
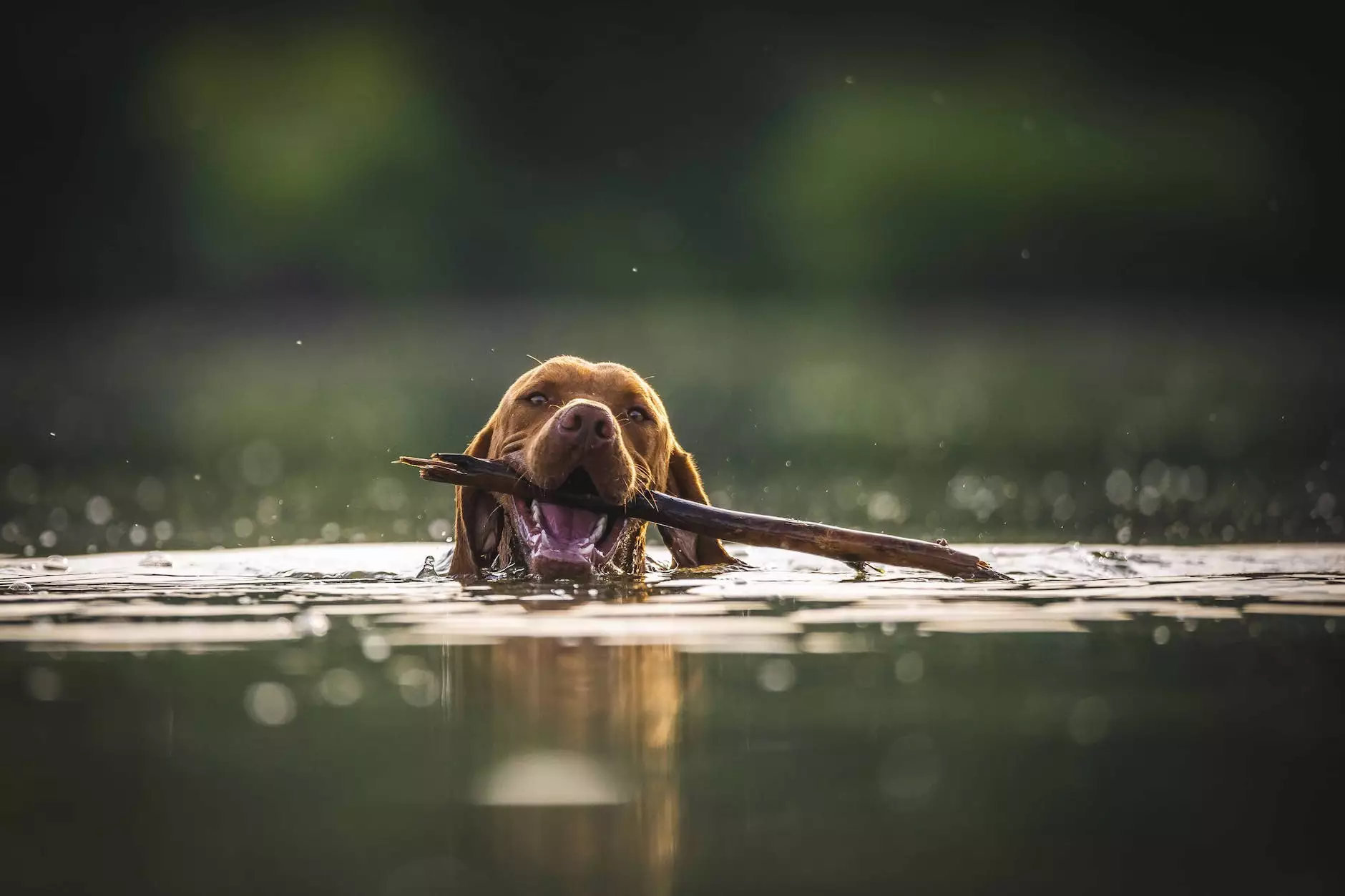
Welcome to the Node.js & Express.js Series by CTIP - The Council for Trade and Investment Promotion. In this chapter, we will explore how to fetch records from the database using Node.js and Express.js.
Introduction
As part of our ongoing series on Node.js and Express.js, we aim to provide comprehensive tutorials that cover various aspects of web development using these technologies. In this chapter, we will focus on fetching records from the database, a crucial step in building dynamic and data-driven web applications.
Why Fetching Records from the Database Matters
Fetching records from the database allows your web application to access and display information stored in a structured manner. It enables you to present real-time data to your users, enhancing their experience and providing them with accurate and up-to-date information.
By using Node.js and Express.js, you can efficiently retrieve records from a database and seamlessly integrate them into your web application. This empowers you to build flexible and responsive websites that adapt to users' needs.
Fetching Records with Node.js & Express.js
To fetch records from a database, you need to establish a connection between Node.js, Express.js, and the chosen database management system (DBMS). Common DBMS options include MySQL, PostgreSQL, MongoDB, and SQLite.
Once the connection is established, you can issue queries to retrieve specific records or perform advanced data manipulation operations. Node.js and Express.js provide robust libraries and frameworks that simplify the process and optimize performance.
Step 1: Setting up the Database Connection
Before fetching records, you need to set up the database connection in your Node.js and Express.js application. This involves installing the necessary database drivers, configuring the connection string, and handling any authentication requirements.
For example, when working with MySQL, you can use the "mysql" package to establish a connection:
const mysql = require('mysql'); const connection = mysql.createConnection({ host: 'localhost', user: 'your_username', password: 'your_password', database: 'your_database' }); connection.connect();Remember to replace "your_username," "your_password," and "your_database" with your actual database credentials.
Step 2: Writing the Query
After establishing the database connection, you can write a query to fetch the records you need. The query syntax varies depending on the DBMS you are using, but they generally follow a similar structure.
For instance, to retrieve all records from a table named "users," you can use the following SQL query:
SELECT * FROM users;Step 3: Executing the Query
Once your query is ready, you can execute it using the appropriate Node.js and Express.js database library. The library will handle sending the query to the DBMS, fetching the result set, and returning the data to your application.
Building upon the previous MySQL example, you can execute a SELECT query and log the results as follows:
connection.query('SELECT * FROM users;', function (error, results, fields) { if (error) throw error; console.log(results); });This code snippet retrieves all records from the "users" table and logs them to the console. You can customize the query to fetch specific records or apply filters based on your application's requirements.
Conclusion
In this chapter of our Node.js & Express.js series, we have explored how to fetch records from a database using Node.js and Express.js. This step is essential for building dynamic and data-driven web applications that provide users with real-time information.
By leveraging the power of Node.js and Express.js, you can efficiently retrieve data from databases and integrate it seamlessly into your web applications. This empowers you to create highly responsive and personalized experiences for your users.
Stay tuned for the next chapter in our series, where we will delve further into advanced database operations and explore more ways to enhance your web development skills using Node.js and Express.js.